지구는 구형태를 띄고 있기 때문에 위치마다 경도 1º, 위도 1º의 거리는 서로 다릅니다. 이를 계산하기 위해서 두 지점의 경도와 위도를 가지고 거리를 계산하는 방법은 "하버사인 공식(Haversine formula)"이라는 수식을 사용합니다. 이 공식은 두 지점의 위도, 경도, 지구 반지름 등을 이용하여 구할 수 있습니다.
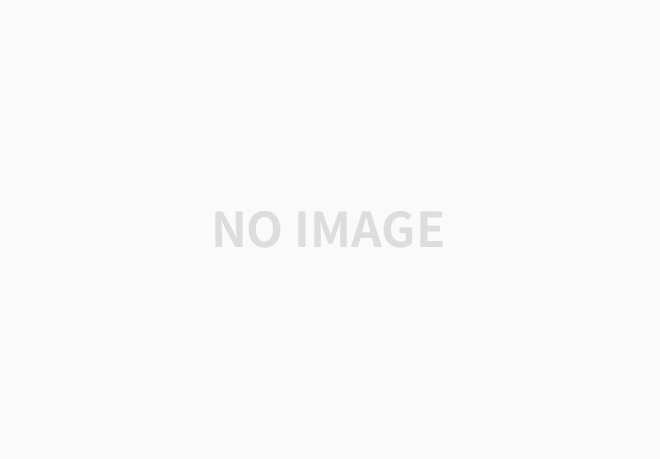
하버사인의 공식은 아래의 링크를 참고하시기 바랍니다.
Haversine formula - Wikipedia
From Wikipedia, the free encyclopedia Formula for the great-circle distance between two points on a sphere The haversine formula determines the great-circle distance between two points on a sphere given their longitudes and latitudes. Important in navigati
en.wikipedia.org
아래는 JavaScript로 구현된 예시 코드입니다.
function distance(lat1, lon1, lat2, lon2) {
const R = 6371; // 지구 반지름 (단위: km)
const dLat = deg2rad(lat2 - lat1);
const dLon = deg2rad(lon2 - lon1);
const a = Math.sin(dLat/2) * Math.sin(dLat/2) +
Math.cos(deg2rad(lat1)) * Math.cos(deg2rad(lat2)) *
Math.sin(dLon/2) * Math.sin(dLon/2);
const c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1-a));
const distance = R * c; // 두 지점 간의 거리 (단위: km)
return distance;
}
function deg2rad(deg) {
return deg * (Math.PI/180);
}
// 예시: 서울과 부산 간의 거리를 계산합니다.
const seoulLat = 37.5665;
const seoulLon = 126.9780;
const busanLat = 35.1796;
const busanLon = 129.0756;
const dist = distance(seoulLat, seoulLon, busanLat, busanLon);
console.log(dist); // 출력: 325.4961911409085
위 코드에서 distance() 함수는 두 지점의 위도(lat1, lat2)와 경도(lon1, lon2)를 인자로 받아서 거리를 계산하여 반환합니다. deg2rad() 함수는 각도를 라디안으로 변환하는 함수입니다. R은 지구 반지름으로, 이 예제에서는 단위를 km로 사용하였습니다.
댓글